- (25 points)
Write the following function that reads a two-dimensional array from file
hw6_1.dat (create this file by yourself and add it to the project) and tests
whether it has four consecutive numbers of the same
value, either horizontally, vertically, or diagonally.
public static boolean isConsecutiveFour(int[][] a)
Your program should print True if the array contains four consecutive
numbers with the same value. Otherwise, display False. Here are some examples
of the true cases:

Your program must work for any array, not just for the above examples.
Name the project Hw6_1
Solution
- (25 points)
Design a Java application to implement the following algorithm for
sorting an array of integers.
According to this algorithm, the sort is accomplished by repeatedly taking
the next item from the array (pivot) and inserting it into the final array
in its proper order with respect to the items already inserted.
Example:
Given the following array a for sorting,
it is traversed just once, the position of the currently processing pivot
is indicated by "^". In each step the marked pivot is inserted to its
proper place in array b. The resulting sorted array is b.
a: 14 5 7 12 8 b: (empty)
step1: 14 5 7 12 8 b: 14
^
step2: 14 5 7 12 8 b: 5 14
^
step3: 14 5 7 12 8 b: 5 7 14
^
step4: 14 5 7 12 8 b: 5 7 12 14
^
step5: 14 5 7 12 8 b: 5 7 8 12 14
^
Your program should contain method int[] inSort(int[]) that takes
the original array (array a in the above example) as a parameter
and returns the sorted array (array b in the above example).
The original and sorted arrays must be printed out.
Name the project Hw6_2
Solution
- (25 points)
Design a class named MyInteger. The class must include the following features:
- A private class variable named value that stores the integer value
represented by this object.
- A constructor that creates MyInteger object for the specified int
value.
- A get method that returns the int value.
- Methods isEven(), isOdd(), and isPrime() that return true if the
value is even, odd, or prime, respectively.
- Static methods isEven(int), isOdd(int), and isPrime(int) that return
true if the specified value is even, odd, or prime, respectively.
- Static methods isEven(MyInteger), isOdd(MyInteger), and
isPrime(MyInteger) that return true if the specified value is even, odd, or
prime, respectively.
- Methods equals(int) and equals(MyInteger) that return true if the
value in the object is equal to the one specified by the parameter.
- A static method parseInt(char[]) that converts an array of numeric
characters to an int value.
- A static method parseInt(String) that converts a string into an int
value.
Implement the class. Write another class with main() method
that would ask the user to enter an integer, instantiate class MyInteger
based on that number, and test all methods in MyInteger class.
Name the project Hw6_3
Solution
- (25 points)
Define the Circle2D class that contains:
- Two private class variables of type double named x and y that specify
the center of the circle with the corresponding public get methods.
- A private field radius its get method.
- A no-argument constructor that creates a default circle with (0, 0) for
(x, y) and 1 for radius.
- A constructor that creates a circle with the specified x, y, and
radius.
- A method getArea() that returns the area of the circle.
- A method getPerimeter() that returns the perimeter of the circle.
- A method contains(double x, double y) that returns true if the
specified point (x, y) is inside this circle. See Fig. (a) below.
- A method contains(Circle2D circle) that returns true if the specified
circle is inside this circle. See Fig. (b) below.
- A method overlaps(Circle2D circle) that returns true if the specified
circle overlaps with this circle. See Fig. (c) below.
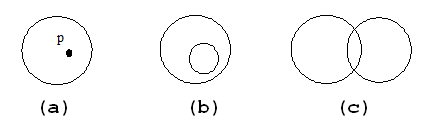
Implement the class. Write a test program that creates a Circle2D object
c1 (new Circle2D(2, 2, 5.5)), displays its area and perimeter, and displays
the result of c1.contains(3, 3), c1.contains(new Circle2D(4, 5, 10.5)), and
c1.overlaps(new Circle2D(3, 5, 2.3)).
Name the project Hw6_4
Solution